Exercise 3 : Entity Control
Description
In this exercise, you will implement a couple of simple behaviors, work with the Unity Input System, and implement local multiplayer.
In case of any problems, please see the FAQ and the Problems and Solutions. If your problem is not listed, contact me by e-mail (Tomas Polasek : ).
Instructions
This exercise consists of three segments. It is recommended to follow the order:
- Gun and Enemy Behavior
- Alternative Control Scheme
- Local Multiplayer
Download the project template from the Materials section and follow the same general procedure as in the second exercise. However, this time, do not use the 2D view, since the template is built in 3D. The primary control scheme already implemented within the game is following:
- WASD : Movement
- Mouse : Aiming
- Left Mouse Button : Shoot
- Space : Switch Mode of Fire
Gun and Enemy Behavior
Start by implementing the Gun behavior by modifying the Scripts/Game/Gun.cs script. First, start up the game and try combination of moving and shooting. Notice, that the bullets doe not originate within the gun, but are instead spawned at the origin. Your goal will be to ammend this problem and implement a secondary mode of fire.
The primary code should be contained within the ShootGun method starting on line 187. First modify the script so that the bullets are correctly oriented and positioned even for moving players. Then, follow by creating a second mode for the weapon by using the shotgun switch (activated by <SPACE> by default). The second mode does not necessarily need to be a real shotgun, but it should be sufficiently different from the main mode of the weapon.
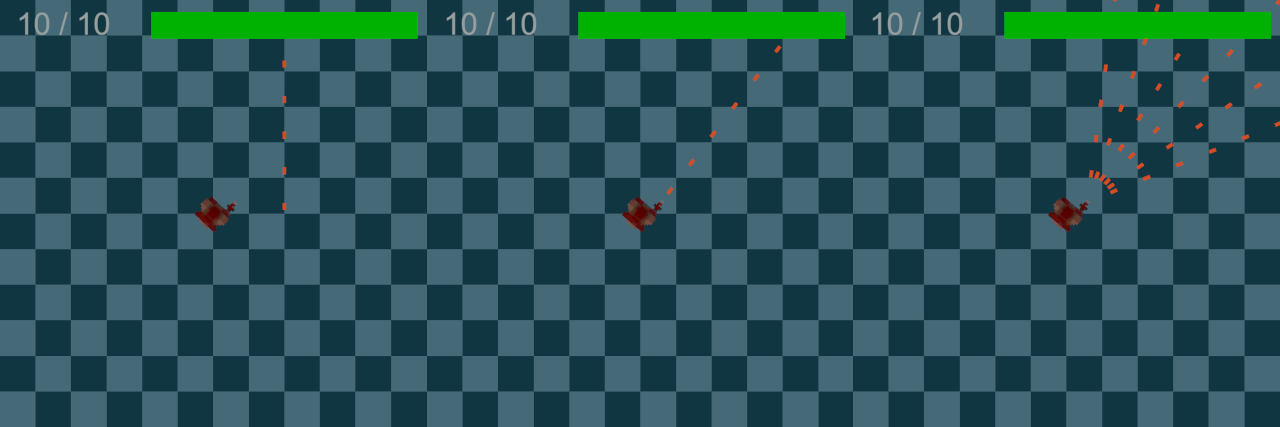
When you get the weapon to work (you will need it), continue with implementing the Enemy behavior. First, enable the spawners already placed within the scene (EnemySpawner and MultipleSpawners). Notice, that the enemies spawned currently do nothing interesting. Your goal will be to make the enemies follow the player. Implement a simple AI which will follow the closest player, by modifying the FixedUpdate method in the Scripts/Game/Enemy.cs script, on line 60.
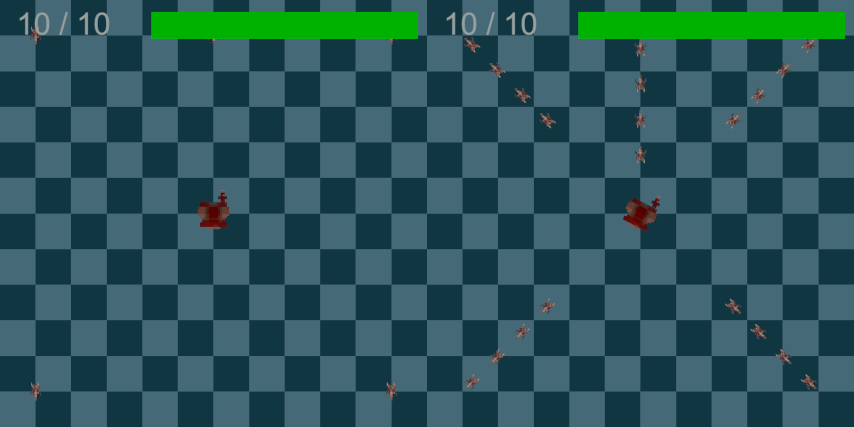
Alternative Control Scheme
To create an alternative control scheme usable by the main character, you will need to modify the default Action Mapping in Input/PlayerInput.inputactions. Open the mapping and start by creating a secondary control scheme. Then, add secondary bindings for each of the pimary actions, using one of the following:
- Left Stick : Movement
- Right Stick : Aiming
- South Button | Right Trigger : Shoot
- North Button : Switch Mode of Fire
In case you don’t have a controller on hand, you can use the virtual controls provided under the Canvas GameObject. To enable the virtual controls, select the VirtualController and enable the GameObject by using the checkbox in the Inspector. Alternatively, you can also utilize different area of the keyboard - e.g. UHJK for movement.
Finally, make sure that the PlayerInput Component on the Prefabs/Player.prefab is correctly set to accept any of the available schemes and auto-switching is enabled.
In case of problems, try re-importing all assets from the right-click menu in the Project/Assets window.
Local Multiplayer
Finally, you will modify the game so that the newly added control scheme does not work as an alternative means of controling the main player, but instead allows multiple players to play at the same time.
Start by adding the Player Input Manager Component to the scene. The addition and removal of the players is handled automatically. However, if you want to detect when a new player joints or leaves, see the main Settings script Scripts/Game/Settings.cs line 100. The second player should appear once any button in the secondary control scheme is used. For further hints, consult the the Unity Documentation on the subject.
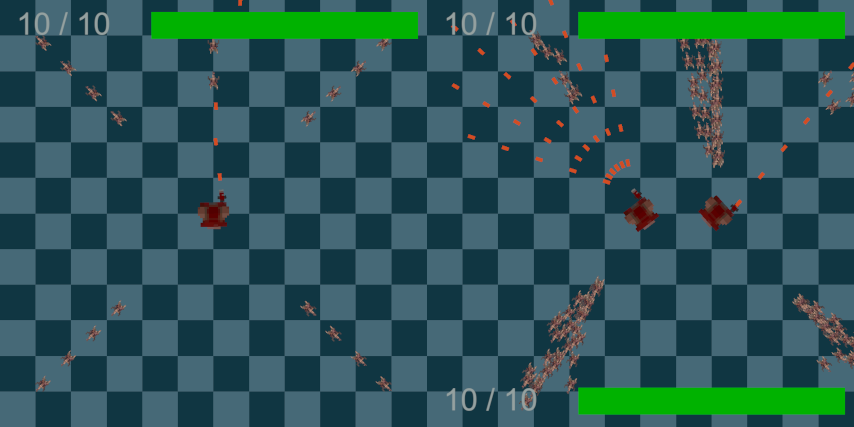
Submit the Exercise
Submit the results according to the procedure detailed in the Exercise Submission chapter.
Task checklist:
- Implement the Gun and Enemy behavior
- Add a secondary Gamepad control scheme
- Allow local multiplayer with second player
FAQ
Following are some problems and solutions which may occur during this exercise:
- In case of input-related errors, try reloading assets (Ctrl + R) or using the Reimport All function from the right-click menu in the project assets.
Materials
Project Template : [zip]